imucal.FerrarisCalibrationInfo#
- class imucal.FerrarisCalibrationInfo(acc_unit: str = 'm/s^2', gyr_unit: str = 'deg/s', from_acc_unit: str | None = None, from_gyr_unit: str | None = None, comment: str | None = None, K_a: ndarray | None = None, R_a: ndarray | None = None, b_a: ndarray | None = None, K_g: ndarray | None = None, R_g: ndarray | None = None, K_ga: ndarray | None = None, b_g: ndarray | None = None)[source]#
Calibration object that represents all the required information to apply a Ferraris calibration to a dataset.
- Parameters:
- K_a
Scaling matrix for the acceleration
- R_a
Rotation matrix for the acceleration
- b_a
Acceleration bias
- K_g
Scaling matrix for the gyroscope
- R_g
Rotation matrix for the gyroscope
- K_ga
Influence of acceleration on gyroscope
- b_g
Gyroscope bias
- Attributes:
- K_a
- K_g
- K_ga
- R_a
- R_g
- b_a
- b_g
- comment
- from_acc_unit
- from_gyr_unit
Methods
calibrate
(acc, gyr, acc_unit, gyr_unit)Calibrate the accelerometer and the gyroscope.
calibrate_df
(df, acc_unit, gyr_unit[, ...])Apply the calibration to data stored in a dataframe.
find_subclass_from_cal_type
(cal_type)Get a SensorCalibration subclass that handles the specified calibration type.
from_hdf5
(path)Read calibration data stored in hdf5 fileformat (created by
CalibrationInfo.save_to_hdf5
).from_json
(json_str)Create a calibration object from a json string (created by
CalibrationInfo.to_json
).from_json_file
(path)Create a calibration object from a valid json file (created by
CalibrationInfo.to_json_file
).to_hdf5
(path)Save calibration matrices to hdf5 file format.
to_json
()Convert all calibration matrices into a json string.
to_json_file
(path)Dump acc calibration matrices into a file in json format.
- __init__(acc_unit: str = 'm/s^2', gyr_unit: str = 'deg/s', from_acc_unit: str | None = None, from_gyr_unit: str | None = None, comment: str | None = None, K_a: ndarray | None = None, R_a: ndarray | None = None, b_a: ndarray | None = None, K_g: ndarray | None = None, R_g: ndarray | None = None, K_ga: ndarray | None = None, b_g: ndarray | None = None) None #
- calibrate(acc: ndarray, gyr: ndarray, acc_unit: str | None, gyr_unit: str | None) Tuple[ndarray, ndarray] [source]#
Calibrate the accelerometer and the gyroscope.
- This corrects:
acc: scaling, rotation, non-orthogonalities, and bias gyro: scaling, rotation, non-orthogonalities, bias, and acc influence on gyro
- Parameters:
- acc
3D acceleration
- gyr
3D gyroscope values
- acc_unit
The unit of the acceleration data
- gyr_unit
The unit of the gyroscope data
- Returns:
- Corrected acceleration and gyroscope values
- calibrate_df(df: DataFrame, acc_unit: str | None, gyr_unit: str | None, acc_cols: Iterable[str] = ('acc_x', 'acc_y', 'acc_z'), gyr_cols: Iterable[str] = ('gyr_x', 'gyr_y', 'gyr_z')) DataFrame [source]#
Apply the calibration to data stored in a dataframe.
This calls
calibrate
for the respective columns and returns a copy of the df with the respective columns replaced by their calibrated counter-part.See the
calibrate
method for more information.- Parameters:
- df
6 column dataframe (3 acc, 3 gyro)
- acc_cols
The name of the 3 acceleration columns in order x,y,z.
- gyr_cols
The name of the 3 acceleration columns in order x,y,z.
- acc_unit
The unit of the acceleration data
- gyr_unit
The unit of the gyroscope data
- Returns:
- cal_df
A copy of
df
with the calibrated data.
- classmethod find_subclass_from_cal_type(cal_type)[source]#
Get a SensorCalibration subclass that handles the specified calibration type.
- classmethod from_hdf5(path: str | Path)[source]#
Read calibration data stored in hdf5 fileformat (created by
CalibrationInfo.save_to_hdf5
).- Parameters:
- path
Path to the hdf5 file
- Returns:
- cal_info
A CalibrationInfo object. The exact child class is determined by the
cal_type
key in the json string.
- classmethod from_json(json_str: str) CalInfo [source]#
Create a calibration object from a json string (created by
CalibrationInfo.to_json
).- Parameters:
- json_str
valid json string object
- Returns:
- cal_info
A CalibrationInfo object. The exact child class is determined by the
cal_type
key in the json string.
- classmethod from_json_file(path: str | Path) CalInfo [source]#
Create a calibration object from a valid json file (created by
CalibrationInfo.to_json_file
).- Parameters:
- path
Path to the json file
- Returns:
- cal_info
A CalibrationInfo object. The exact child class is determined by the
cal_type
key in the json string.
Examples using imucal.FerrarisCalibrationInfo
#
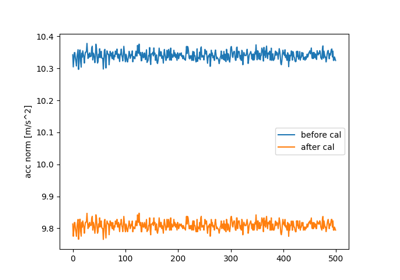
Annotate a session and perform a Ferraris Calibration